Technical Skills Required for Artificial Intelligence
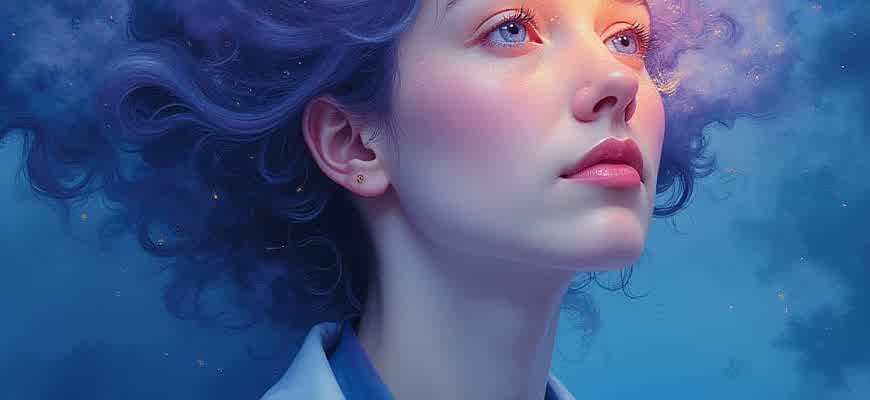
Artificial Intelligence (AI) development requires a diverse set of technical skills, ranging from programming expertise to deep understanding of algorithms. Professionals working in AI need to be equipped with both theoretical knowledge and practical tools to build efficient models and systems. The following areas are critical for anyone pursuing a career in AI.
Programming Languages play a crucial role in AI. Mastery of languages like Python, R, and C++ is fundamental for implementing AI algorithms and handling data efficiently.
- Python is the most widely used language due to its simplicity and vast libraries such as TensorFlow, Keras, and PyTorch.
- R is favored for statistical computing and data analysis, making it useful in machine learning tasks.
- C++ is important for performance-heavy applications, particularly in areas requiring real-time processing.
Mathematics and Statistics form the foundation of AI algorithms. Knowledge of linear algebra, probability theory, and calculus is necessary to understand how AI models function and improve over time.
AI practitioners must have an understanding of optimization techniques, matrix operations, and data distributions to implement machine learning models effectively.
Machine Learning and Data Science are core domains in AI. Proficiency in machine learning algorithms, including supervised, unsupervised, and reinforcement learning, is a must. Data preprocessing, feature engineering, and model evaluation are integral parts of the process.
- Supervised learning methods such as decision trees, SVMs, and regression models.
- Unsupervised learning algorithms, including clustering techniques like K-means and dimensionality reduction methods like PCA.
- Reinforcement learning approaches to train agents in dynamic environments.
Tools and Frameworks are essential to streamline the development process. Familiarity with libraries such as TensorFlow, Keras, and PyTorch allows AI practitioners to build and deploy models effectively.
Tool | Purpose |
---|---|
TensorFlow | Open-source library for numerical computation and machine learning. |
Keras | High-level neural networks API, often used with TensorFlow. |
PyTorch | Deep learning library known for flexibility and speed in research. |
Mastering Python for AI Development
Python has become a dominant programming language in artificial intelligence (AI) development due to its simplicity, readability, and extensive library support. To effectively leverage Python for AI projects, developers must be familiar with a set of core skills that enable them to implement algorithms, manage data, and build AI systems. These include understanding data structures, using AI libraries, and optimizing code for performance. Mastery of Python goes beyond basic syntax, involving a deep understanding of its ecosystem and how to efficiently apply it to AI tasks.
To develop expertise in Python for AI, one must not only understand the syntax but also know how to utilize powerful libraries such as NumPy, Pandas, and TensorFlow. Additionally, proficiency in machine learning frameworks and the ability to work with large datasets are crucial. A clear understanding of Python’s object-oriented programming (OOP) features also plays an essential role in structuring complex AI solutions.
Core Skills for AI Development in Python
- Data Manipulation: Efficient handling of data with Pandas and NumPy for preprocessing and transformation tasks.
- Machine Learning Libraries: Mastery of Scikit-learn, TensorFlow, and PyTorch to implement algorithms and build models.
- Algorithms and Models: Understanding the inner workings of machine learning models like decision trees, neural networks, and reinforcement learning.
- Deep Learning Frameworks: Familiarity with advanced tools such as Keras and PyTorch for developing deep learning models.
"The key to mastering Python for AI is not just writing code but understanding how libraries interact to solve complex problems efficiently."
Key Python Libraries for AI Development
Library | Purpose |
---|---|
NumPy | Used for numerical operations and handling large multi-dimensional arrays. |
Pandas | Provides data structures for efficient data manipulation and analysis. |
TensorFlow | A deep learning framework for developing neural networks and complex AI systems. |
Scikit-learn | Used for implementing machine learning algorithms and preprocessing data. |
PyTorch | Offers tools for building and training deep learning models, with dynamic computation graphs. |
Important Concepts for AI Development in Python
- Object-Oriented Programming (OOP): Essential for creating modular and maintainable AI code.
- Data Structures: Lists, dictionaries, sets, and arrays are fundamental for efficient data handling.
- Vectorization: Optimizing code performance by replacing loops with vectorized operations using libraries like NumPy.
- Parallel Computing: Using multi-threading and GPU-based computation to speed up complex AI tasks.
Key Data Structures and Algorithms for AI Problem-Solving
In the field of Artificial Intelligence, the selection of the appropriate data structures and algorithms is critical for building efficient models and solving complex problems. AI tasks, such as machine learning, computer vision, and natural language processing, often require efficient ways to process and store large datasets. Understanding the fundamentals of data structures and algorithms allows AI engineers to optimize performance and reduce computational costs.
Key data structures and algorithms enable AI systems to process and manipulate data effectively, ensuring scalability and accuracy. Below, we discuss some of the most essential data structures and algorithms that play a crucial role in AI problem-solving.
Essential Data Structures
- Arrays – Simple, fixed-size collections of elements that allow quick access to indexed data. Used in storing datasets and in algorithms like searching and sorting.
- Graphs – Structures that consist of nodes and edges, widely used in tasks like network analysis, pathfinding, and recommendation systems.
- Hash Tables – Store data in key-value pairs, enabling fast lookup times, often used in tasks requiring quick retrieval, such as caching and indexing.
- Heaps – Specialized tree-based data structures that maintain a partial order, useful for implementing priority queues in algorithms like A* search and Dijkstra's algorithm.
Common Algorithms for AI
- Search Algorithms – Essential for exploring solution spaces, such as breadth-first search (BFS) and depth-first search (DFS), used in pathfinding and AI planning tasks.
- Sorting Algorithms – Algorithms like quick sort and merge sort help in organizing data, often required for preprocessing in machine learning pipelines.
- Dynamic Programming – Optimizes problems by breaking them down into subproblems and storing solutions, often used in reinforcement learning and optimization tasks.
Important Note: Mastery of data structures and algorithms is foundational to designing efficient AI models. Without an understanding of how to manipulate data efficiently, even the most sophisticated algorithms can fail to deliver optimal results.
Comparison of Algorithms in AI
Algorithm | Application | Time Complexity |
---|---|---|
Quick Sort | Efficient sorting for large datasets | O(n log n) |
A* Search | Pathfinding, navigation | O(b^d) |
Dynamic Programming | Optimization, sequence alignment | O(n) |
Understanding Machine Learning Frameworks and Libraries
Machine learning frameworks and libraries are essential tools that simplify the development and deployment of machine learning models. These resources provide predefined structures and functions, allowing developers to focus on model design rather than low-level implementation details. They offer a range of functionalities, including data preprocessing, model building, and evaluation. With their ease of use and extensive documentation, these tools have become indispensable for both beginners and experienced professionals in the field of AI.
The choice of framework or library can significantly impact the efficiency, performance, and scalability of a machine learning project. Frameworks typically offer high-level APIs for model development, while libraries may provide more specialized tools for specific tasks like data manipulation or visualization. Below, we will explore some popular machine learning frameworks and libraries that are widely used in AI development.
Key Machine Learning Frameworks
- TensorFlow: Developed by Google, TensorFlow is a powerful open-source library for numerical computation and machine learning. It supports deep learning, neural networks, and large-scale data analysis, making it ideal for both research and production environments.
- PyTorch: Known for its dynamic computation graph and ease of use, PyTorch is widely adopted in the research community. It is highly flexible, making it suitable for rapid prototyping and experimentation with complex neural network architectures.
- scikit-learn: A versatile library for classical machine learning algorithms, scikit-learn supports tasks like classification, regression, and clustering. It is widely used for smaller-scale projects and is an excellent choice for those starting with machine learning.
Popular Libraries for Specific ML Tasks
- Pandas: A powerful library for data manipulation and analysis, widely used for preprocessing tasks such as cleaning, transforming, and merging datasets.
- NumPy: Essential for numerical computations, NumPy provides an array object that is efficient for large datasets and supports operations on matrices and vectors.
- Matplotlib: A plotting library used for visualizing data and results, essential for analyzing the performance of machine learning models.
Frameworks Comparison
Framework | Strengths | Use Cases |
---|---|---|
TensorFlow | Scalability, production-ready, extensive documentation | Deep learning, large-scale systems, cross-platform deployment |
PyTorch | Dynamic computation, flexibility, easy debugging | Research, experimentation, rapid prototyping |
scikit-learn | Ease of use, integration with other libraries | Traditional machine learning algorithms, smaller datasets |
Important: The choice of machine learning framework should align with the project requirements, such as scalability, ease of use, or the need for rapid experimentation. For large-scale production systems, TensorFlow is often preferred, while PyTorch is the go-to option for research-oriented tasks.
Deep Learning: Neural Networks and Their Applications
Deep learning is a subfield of machine learning that focuses on algorithms inspired by the structure and functioning of the human brain. It primarily involves the use of neural networks, which consist of layers of interconnected nodes, or neurons, that process and analyze vast amounts of data. These networks are capable of learning complex patterns from raw data, making them highly effective in tasks like image recognition, natural language processing, and autonomous driving.
Neural networks can be divided into several types, each suited for specific applications. The most common types include feedforward networks, convolutional neural networks (CNNs), and recurrent neural networks (RNNs). These architectures are highly customizable, enabling engineers to create solutions tailored to a wide variety of industries, from healthcare to finance.
Key Neural Network Architectures
- Feedforward Neural Networks (FNNs): Basic architecture used for tasks like classification and regression.
- Convolutional Neural Networks (CNNs): Specialize in image-related tasks by using layers that can identify patterns in visual data.
- Recurrent Neural Networks (RNNs): Suitable for sequential data like time series and natural language processing.
Applications of Neural Networks
- Image Recognition: Used in applications like facial recognition and medical image analysis.
- Natural Language Processing: Enables machines to understand and generate human language, used in chatbots and language translation.
- Autonomous Vehicles: Neural networks power self-driving cars by helping them interpret sensory data in real-time.
"Neural networks have revolutionized industries by offering a powerful way to analyze data and make predictions with high accuracy."
Comparison of Neural Network Architectures
Architecture | Strengths | Applications |
---|---|---|
Feedforward Neural Networks | Simple and fast; good for basic classification tasks. | Classification, Regression |
Convolutional Neural Networks | Excellent for image processing; detects spatial hierarchies. | Image Recognition, Video Analysis |
Recurrent Neural Networks | Handles sequential data well; preserves temporal information. | Speech Recognition, Text Generation |
Grasping Natural Language Processing Techniques
Natural Language Processing (NLP) is a key component in the development of intelligent systems that understand and generate human language. Understanding NLP techniques requires a solid foundation in both computational linguistics and machine learning. Professionals in this field must be equipped with the ability to process text, analyze patterns, and implement algorithms that enable machines to interpret natural language effectively.
The core of NLP involves breaking down language into units such as tokens, sentences, and semantic structures. Mastery of techniques such as tokenization, part-of-speech tagging, and named entity recognition is essential. NLP specialists must also comprehend the application of machine learning models like decision trees, neural networks, and transformers for tasks ranging from translation to sentiment analysis.
Key NLP Techniques
- Tokenization: Dividing text into smaller units, like words or phrases, to simplify processing.
- Part-of-speech Tagging: Assigning grammatical labels to words to understand their syntactic roles.
- Named Entity Recognition: Identifying and classifying named entities (e.g., people, organizations) in text.
- Sentiment Analysis: Determining the emotional tone behind a body of text.
- Machine Translation: Automatically translating text from one language to another.
Machine Learning Models for NLP
- Recurrent Neural Networks (RNNs): Excellent for sequential data, making them effective in language modeling and text generation.
- Transformers: A breakthrough in NLP, enabling models like BERT and GPT to process and generate context-rich text.
- Decision Trees: Useful for simple NLP tasks like text classification and categorization.
- Support Vector Machines (SVM): Efficient for classification tasks, particularly in sentiment analysis.
"To truly master NLP, one must not only understand algorithms but also the intricacies of human language and its various nuances."
Comparison of NLP Models
Model | Strengths | Limitations |
---|---|---|
RNN | Good for sequential data processing | Vanishing gradient problem |
Transformer | High performance in large-scale tasks | Requires significant computational resources |
SVM | Effective for small to medium datasets | Not ideal for handling large-scale text data |
Familiarity with Big Data and Distributed Computing for AI
Artificial Intelligence (AI) systems often require the processing of vast datasets, which necessitates knowledge of Big Data technologies. Big Data refers to datasets that are too large or complex to be processed using traditional data-processing techniques. To leverage the full potential of AI, practitioners need expertise in handling these datasets efficiently. Distributed computing plays a critical role in this process, enabling the distribution of tasks across multiple machines, improving processing speed and system scalability.
In addition to understanding data storage and processing techniques, familiarity with distributed computing frameworks allows AI developers to handle large-scale computations that AI models require. Whether it's for training deep learning algorithms or processing massive amounts of real-time data, distributed computing infrastructures ensure AI systems can scale and perform efficiently. This integration is essential for systems that demand continuous learning and real-time predictions.
Key Concepts in Big Data and Distributed Computing for AI
- Data Processing Frameworks: Tools like Hadoop and Apache Spark allow the processing of large data sets across distributed systems. These frameworks are crucial for AI models that require parallel processing.
- Cloud Computing Platforms: Cloud services like AWS, Azure, and Google Cloud provide the infrastructure needed for scalable AI applications, supporting both data storage and computational power.
- Data Storage Solutions: Systems such as NoSQL databases (e.g., MongoDB, Cassandra) and distributed file systems (e.g., HDFS) are designed to handle Big Data storage needs, which is essential for AI model training.
Distributed Computing Tools for AI
- Apache Spark: A widely used distributed computing framework for handling large-scale data processing, including machine learning algorithms.
- TensorFlow on Kubernetes: A popular AI framework that can be deployed on distributed computing clusters for faster model training and execution.
- MapReduce: A programming model that allows the parallel processing of data across multiple nodes, commonly used for Big Data tasks in AI.
Important: Distributed computing is not only about parallelizing tasks but also ensuring data consistency and fault tolerance, which are crucial for maintaining the integrity of AI model training.
Comparison of Distributed Computing Technologies
Framework | Key Feature | Best Use Case |
---|---|---|
Hadoop | Batch processing, fault tolerance | Large-scale data storage and batch processing |
Apache Spark | Real-time processing, in-memory computing | Machine learning algorithms, real-time data analytics |
TensorFlow on Kubernetes | AI model training, scalable deployment | Distributed deep learning and AI applications |
Mathematics Behind AI: Linear Algebra, Probability, and Statistics
In the field of artificial intelligence (AI), a strong foundation in mathematics is essential for understanding and developing complex algorithms. AI leverages several mathematical principles to process and interpret data efficiently, enabling systems to "learn" from input and make informed decisions. Three key areas of mathematics that play a central role in AI are linear algebra, probability theory, and statistics.
Linear algebra provides the necessary tools for handling data structures such as vectors and matrices, which are essential for many machine learning algorithms. Probability theory enables the modeling of uncertainty, while statistics helps in drawing insights from data and making predictions. Below is a breakdown of the importance of each of these mathematical areas.
Linear Algebra
Linear algebra is the backbone of many machine learning algorithms, particularly in deep learning and neural networks. Key concepts include:
- Vectors: Represent data points in a multidimensional space.
- Matrices: Allow efficient storage and transformation of data, enabling operations on large datasets.
- Eigenvalues and Eigenvectors: Essential for understanding transformations and optimizations in data processing.
These tools are used to manipulate and transform data, and they are the basis for many operations in AI models.
Probability Theory
Probability is critical for AI models that need to make predictions based on uncertain or incomplete data. Key concepts include:
- Bayes' Theorem: Helps in updating the probability of a hypothesis based on new evidence.
- Conditional Probability: Used in probabilistic models like Bayesian networks to understand dependencies between variables.
- Random Variables: Represent outcomes that are subject to chance, crucial for simulating and understanding uncertainties in AI systems.
Understanding and applying probability allows AI systems to handle ambiguity and make decisions even when the input data is not certain.
Statistics
Statistics plays a pivotal role in analyzing data and drawing conclusions. In AI, it is primarily used for:
- Data Analysis: Summarizing large datasets to identify patterns and trends.
- Hypothesis Testing: Determining the validity of assumptions and models used in AI systems.
- Regression Analysis: Modeling relationships between variables to predict future outcomes.
Statistics is used not only to validate AI models but also to optimize their performance by identifying biases and errors in predictions.
Comparison of Key Concepts in AI Mathematics
Mathematical Area | Key Concepts | Applications in AI |
---|---|---|
Linear Algebra | Vectors, Matrices, Eigenvalues, Eigenvectors | Data transformation, Neural networks, Deep learning |
Probability Theory | Bayes' Theorem, Conditional Probability, Random Variables | Predictive modeling, Decision-making under uncertainty |
Statistics | Data Analysis, Hypothesis Testing, Regression | Pattern recognition, Model optimization, Error analysis |