Adaptive Learning Python
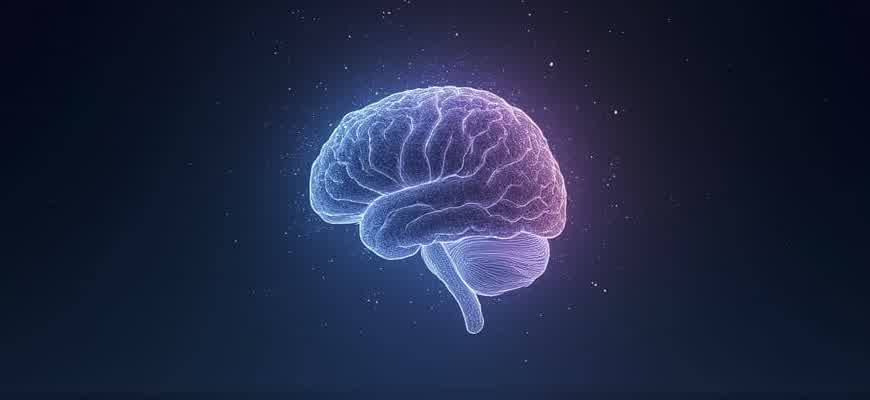
Adaptive learning in Python refers to techniques and methods that allow a system to dynamically adjust its learning path based on the user's progress. This personalized approach optimizes the learning experience by tailoring content to the learner's needs and performance. It's widely used in e-learning platforms, educational software, and skill development tools.
To implement adaptive learning, Python developers rely on several core components:
- Data collection: Gathering user interaction data, including time spent on tasks and correctness of answers.
- Performance analysis: Evaluating the learner's strengths and weaknesses through algorithms.
- Content adjustment: Modifying the learning material or pacing based on real-time analysis.
Key considerations in adaptive learning systems:
Factor | Importance |
---|---|
Scalability | Systems must handle increasing numbers of users without losing performance. |
Real-time feedback | Instant updates on progress help keep the learner engaged and informed. |
"Adaptive learning in Python is not just about teaching concepts but fostering an environment where each user experiences learning in the most effective way for them."
How Adaptive Learning Adjusts to Individual Learning Styles in Python
Adaptive learning systems tailor educational experiences based on the learner's preferences and proficiency. In Python programming, this approach ensures that learners can progress at their own pace while receiving content suited to their understanding and style. The system collects data on the learner's interactions, and adjusts the complexity of tasks and explanations accordingly. This helps to create a more personalized, efficient, and engaging learning environment.
When applied to Python, adaptive learning can modify its teaching methods based on factors like how quickly a learner grasps concepts, how often they need repetition, or their preferred mode of learning–visual, auditory, or hands-on. The key to this adaptability lies in the system's ability to assess real-time performance and make adjustments to the curriculum accordingly.
Adapting to Learning Styles
- Visual learners: These learners benefit from interactive diagrams, flowcharts, and visual representations of Python code. The system may adjust by providing visual aids that clarify complex concepts like loops, data structures, or object-oriented principles.
- Auditory learners: For those who absorb information through listening, the system might offer voice-guided explanations or tutorials, focusing on auditory cues and verbal reinforcement of Python concepts.
- Kinesthetic learners: These individuals learn best by doing. Adaptive learning tools in Python can present hands-on coding exercises, interactive coding challenges, or real-time feedback during projects to cater to their practical approach.
Customization of Learning Paths
- Assessment of learner performance: Adaptive systems begin by evaluating the learner’s initial proficiency with Python basics.
- Content adjustment: Based on ongoing performance, content becomes more advanced or provides additional support for areas of struggle.
- Feedback and reinforcement: Immediate feedback is provided for each completed task, ensuring learners understand their mistakes and progress toward mastering Python concepts.
"The key to adaptive learning lies in its ability to create a personalized experience that responds to the learner’s strengths, challenges, and preferences."
Comparison of Adaptive Learning Approaches
Learning Style | Adaptive Learning Method | Example in Python |
---|---|---|
Visual | Provides visual aids and diagrams | Interactive flowcharts for understanding loops |
Auditory | Incorporates voice-guided tutorials | Python podcast explaining functions and modules |
Kinesthetic | Hands-on coding exercises and real-time feedback | Interactive coding challenges on online platforms |
Advantages of Adaptive Learning for Mastering Python Programming
Adaptive learning has become a transformative approach in education, offering tailored experiences that align with individual learning paces and preferences. When applied to Python programming, this method ensures a deeper understanding of the language by adjusting content difficulty based on the learner's current knowledge. By leveraging real-time feedback and personalized pathways, learners can progress efficiently and focus on areas requiring improvement.
This approach not only accelerates learning but also boosts retention, as learners interact with content that adapts to their evolving skills. Adaptive learning for Python programming minimizes frustration caused by outdated or overly complex material, making the learning process more engaging and less overwhelming. Below are key benefits of using adaptive learning in Python programming mastery.
Key Advantages
- Personalized Learning Pathways – Adaptive systems provide custom-tailored content based on the learner's individual performance, ensuring they focus on areas needing improvement and move past concepts they have already mastered.
- Efficient Skill Development – By eliminating unnecessary repetition, learners spend more time on challenging topics and practice those skills until they are proficient.
- Increased Retention Rates – Repetition of key concepts at optimal intervals improves memory retention, helping learners internalize complex Python syntax and programming principles more effectively.
- Real-time Feedback – Adaptive platforms offer immediate feedback, allowing learners to correct mistakes and adjust their approach before reinforcing incorrect understanding.
How Adaptive Learning Enhances Python Programming
- Targeted Focus on Weak Areas – Adaptive systems identify weaknesses in coding skills and provide exercises or tutorials to target specific gaps.
- Scalable Learning Experience – Whether a beginner or advanced learner, adaptive systems adjust to the learner's level, providing suitable challenges as they grow.
- Motivation Through Achievable Milestones – By presenting smaller, incremental learning goals, adaptive learning keeps learners motivated as they see consistent progress in mastering Python.
"Adaptive learning allows learners to focus on their unique journey, providing tailored exercises and real-time feedback that help them excel in mastering Python programming concepts."
Summary Comparison
Traditional Learning | Adaptive Learning |
---|---|
One-size-fits-all approach, no customization. | Personalized content and feedback tailored to the learner’s progress. |
Fixed curriculum, often too fast or slow for individual learners. | Dynamic curriculum that adjusts based on performance and needs. |
Delayed feedback, which can hinder learning. | Immediate feedback, allowing quick corrections and deeper understanding. |
Implementing Adaptive Learning Algorithms in Python Projects
Adaptive learning systems aim to adjust their teaching strategies based on the learner's performance. These algorithms personalize the learning experience by analyzing data and modifying content in real-time. Python, with its rich ecosystem of libraries and tools, is well-suited for building such systems, allowing developers to implement effective and dynamic learning solutions.
To incorporate adaptive learning into Python-based projects, it is essential to understand how these algorithms work, how they assess user progress, and how they deliver personalized feedback. The following steps outline the process of implementing such systems efficiently.
Steps to Implement Adaptive Learning in Python
- Data Collection and Preprocessing: Collect data from users, such as quiz scores, response times, and interactions. Clean and preprocess this data to remove outliers or inconsistencies.
- Performance Evaluation: Develop models that can track learners' progress and performance over time. Metrics such as accuracy, time per task, and repetition rates help assess how well a learner is doing.
- Adaptive Content Delivery: Based on the learner's performance, adapt the content. For instance, if a user struggles with a topic, the system can provide more practice questions or offer additional resources.
- Feedback Loop: Continuously update the model and its recommendations by feeding it new data from the learner's actions. This iterative process helps improve the learning experience.
Popular Libraries for Adaptive Learning
Several Python libraries can facilitate the development of adaptive learning algorithms. These libraries provide a foundation for handling the data processing, machine learning, and user interaction components of adaptive learning systems.
Library | Purpose |
---|---|
Scikit-learn | Used for machine learning models, helping to create adaptive algorithms based on learners' data. |
TensorFlow | Facilitates deep learning models that can improve over time with user interaction. |
Pandas | Ideal for handling and analyzing user data before feeding it into adaptive learning models. |
Adaptive learning systems become more effective as they continuously evolve based on user data, offering a personalized learning experience that enhances retention and mastery of concepts.
Creating Custom Python Models for Personalized Learning Paths
In the realm of adaptive learning, creating custom Python models allows educators and developers to tailor learning experiences to individual needs. By leveraging machine learning algorithms and data-driven insights, personalized learning paths can be constructed that adjust to a learner’s pace, strengths, and weaknesses. This type of model provides a more effective and engaging experience by continuously evolving based on user performance.
Custom models can be designed to analyze various learning parameters, such as topic proficiency, time spent on tasks, and success rates. This approach ensures that learners are provided with content that suits their current skill level, avoiding unnecessary repetition or overwhelming challenges. The following steps outline how to build a personalized learning model using Python.
Steps to Create Custom Python Models for Adaptive Learning
- Data Collection: Gather information on the learner's interactions with the material, such as quiz results, time on tasks, and progress tracking.
- Feature Engineering: Extract relevant features from the collected data to evaluate the learner's strengths and weaknesses.
- Model Development: Choose an appropriate machine learning algorithm (e.g., decision trees, neural networks) to build a model that predicts the learner’s next optimal learning step.
- Feedback Loop: Implement a system for the model to receive real-time feedback based on the learner's progress and adjust the learning path accordingly.
- Testing and Evaluation: Continuously assess the model's effectiveness in guiding learners through a personalized experience.
Example Model Implementation
Here is a simple example of how a Python-based model can be created for adaptive learning using a decision tree algorithm:
from sklearn.tree import DecisionTreeClassifier import pandas as pd # Example data data = {'score': [90, 70, 85, 60, 75], 'time_spent': [30, 45, 25, 50, 40], 'next_topic': ['Advanced', 'Intermediate', 'Advanced', 'Beginner', 'Intermediate']} df = pd.DataFrame(data) # Define features and target X = df[['score', 'time_spent']] y = df['next_topic'] # Train the model model = DecisionTreeClassifier() model.fit(X, y) # Predict the next topic for a new learner new_learner = [[80, 35]] predicted_topic = model.predict(new_learner) print(f"The learner's next topic is: {predicted_topic[0]}")
Key Considerations for Success
Consideration | Impact |
---|---|
Data Quality | High-quality data is crucial for accurate predictions and learning path personalization. |
Algorithm Choice | The choice of algorithm affects the model's ability to adapt and scale effectively to different learners. |
Real-Time Adaptation | Ensuring that the model updates based on ongoing learner performance helps maintain relevance. |
Note: The success of personalized learning models depends on continuous feedback loops to refine and enhance the learning path dynamically.
Optimizing User Progress Tracking with Python in Adaptive Learning Systems
Efficient tracking of user progress is crucial in adaptive learning environments. By using Python's versatile libraries and tools, educational platforms can better understand and respond to individual learners' needs. Python's rich ecosystem offers various methods for data processing, analysis, and visualization, which are essential for maintaining dynamic learning experiences. Leveraging these capabilities helps educators and developers track learning behaviors in real-time, personalize content, and optimize the path towards mastery.
To effectively monitor user progress, it's vital to collect data at multiple touchpoints throughout the learning journey. This data can then be analyzed to assess performance, identify strengths and weaknesses, and dynamically adjust learning materials. The implementation of Python-based tracking systems can allow for detailed insights into student interaction, engagement, and progress within a course or module.
Methods for Tracking User Progress
- Data Collection: Collect user activity data, including quiz results, time spent on tasks, and interaction history.
- Progress Metrics: Measure completion rates, accuracy, and task performance to evaluate user achievement.
- Feedback Mechanisms: Provide real-time feedback based on user behavior, enabling quick adaptations to learning paths.
Python Libraries for Progress Tracking
- Pandas: Efficiently stores and manipulates user data for analysis.
- Matplotlib & Seaborn: Visualizes user performance trends and insights through graphs and charts.
- Scikit-learn: Implements machine learning algorithms to predict and personalize the learning experience.
Key Techniques for Data Analysis
Data analysis plays a pivotal role in refining adaptive learning systems. By applying Python’s data science tools, patterns in user progress can be identified, and learning content can be tailored for individual needs.
Metric | Data Type | Usage |
---|---|---|
Time Spent | Numeric | Assess user engagement and interaction speed. |
Accuracy | Percentage | Measure learning outcomes and task completion efficiency. |
Activity Frequency | Count | Track user consistency and frequency of task interactions. |
Incorporating Interactive Quizzes and Exercises with Python in Personalized Learning Systems
Interactive quizzes and exercises are vital elements in the development of adaptive learning platforms. They provide an engaging way to assess and reinforce learners' progress. In Python, these elements can be easily integrated into the platform to provide real-time feedback, adjust content based on user performance, and create a more customized learning experience.
Python offers a variety of libraries and frameworks for building interactive content, such as Flask or Django for web-based applications. Through dynamic content generation and user-specific pathways, these tools can help create quizzes and exercises that adapt to the learner’s needs and skill level. This allows the system to evaluate performance and adjust future tasks accordingly.
Types of Interactive Content for Adaptive Learning
- Quizzes: Multiple-choice questions, short answer prompts, and coding challenges that test understanding.
- Exercises: Hands-on tasks where learners can practice coding in real-time, such as writing Python functions or solving algorithmic problems.
- Simulations: Scenarios where learners can apply concepts to virtual environments or projects, receiving instant feedback on their approach.
Adaptive Features in Interactive Content
The key feature of adaptive learning is the ability to modify the learning path based on user interactions. This is made possible by leveraging data collected from quizzes and exercises. Some common methods include:
- Difficulty Adjustment: If a learner answers correctly in a series of questions, the system increases the difficulty level of subsequent tasks.
- Personalized Feedback: Providing real-time hints, suggestions, and explanations based on the learner's answers.
- Progress Tracking: Monitoring performance to suggest areas for improvement and guide the learner through a tailored learning journey.
Important: In order to achieve efficient adaptation, it is crucial to track both the learner's responses and the time taken to complete each task. This data informs the system of the learner's pace, enabling precise adjustments to the learning path.
Technical Implementation Example
A simple implementation of an interactive quiz in Python can utilize the following structure:
Step | Description |
---|---|
1 | Use Python to generate questions dynamically based on the learner's progress. |
2 | Capture responses and evaluate correctness using if-else logic or functions. |
3 | Provide feedback based on answers, adjusting the difficulty of next tasks based on performance. |
Measuring Learner Success with Data-Driven Insights in Adaptive Python Systems
In the context of adaptive learning systems, particularly those focusing on Python programming, measuring learner progress is essential to ensuring personalized educational experiences. These systems gather data on learner interactions, engagement, and performance, providing valuable insights for instructors and developers. By analyzing this data, educators can adjust content delivery, tailor challenges, and enhance overall learning outcomes.
Data-driven approaches in adaptive Python learning systems allow for continuous assessment of learner success. Various metrics such as completion rates, error patterns, and time spent on tasks are used to create personalized learning paths. These insights not only help identify areas where learners may need additional support but also allow for predictive adjustments to the learning process, ensuring that students receive the most relevant resources at the right time.
Key Metrics for Success Measurement
- Performance Analytics: Tracks the learner's success in completing coding exercises and tests.
- Engagement Levels: Measures time spent on tasks and frequency of interaction with the system.
- Error Patterns: Identifies recurring mistakes to pinpoint specific areas of difficulty.
Data-Driven Adjustments
- Personalized Content: Content is dynamically adapted to match learner strengths and weaknesses.
- Adaptive Difficulty: System adjusts the complexity of tasks based on learner progress.
- Real-Time Feedback: Learners receive immediate suggestions based on performance data.
Important: Continuous data collection enables the system to dynamically adjust content, offering learners a personalized experience tailored to their needs and pace.
Example Data Representation
Metric | Value |
---|---|
Completion Rate | 85% |
Average Time per Task | 15 minutes |
Error Rate | 10% |
Top Tools and Libraries for Building Adaptive Learning Systems in Python
Building adaptive learning systems requires a blend of machine learning, data analytics, and educational technology. Python provides an array of tools and libraries that facilitate the creation of intelligent, personalized learning environments. These tools can dynamically adjust the difficulty and content based on learner performance, making it crucial for educators and developers to choose the right tools when designing adaptive learning systems.
Python offers numerous libraries that enable the development of adaptive learning systems. These tools leverage artificial intelligence (AI), natural language processing (NLP), and machine learning algorithms to optimize educational content delivery and improve user engagement through personalization. Below are some of the most popular tools and libraries that help in creating robust adaptive learning systems.
Key Libraries and Tools for Adaptive Learning
- TensorFlow: A powerful library for machine learning and deep learning, which is essential for building models that can predict and adapt based on learner behavior and performance.
- Scikit-learn: Ideal for building simple machine learning models. It includes tools for data mining and data analysis, useful for predicting learning patterns and adapting content.
- Keras: A high-level neural networks API, which is highly useful for rapid experimentation and building adaptive learning models with deep learning techniques.
- NLTK: A toolkit for working with human language data (text), useful in adaptive systems that require natural language processing to understand and assess learner inputs.
- OpenAI GPT: Advanced natural language processing models capable of generating personalized learning content and providing real-time feedback to learners.
Libraries for Data Analytics in Adaptive Learning
Effective adaptive learning systems depend on data-driven insights to modify the learning path of each student. Libraries like Pandas and Matplotlib offer powerful tools for data analysis and visualization, enabling developers to identify trends and assess the effectiveness of personalized learning approaches.
"Data analytics is a key factor in optimizing adaptive learning experiences by providing actionable insights into the learner's progress."
Example of Popular Adaptive Learning Tools
Tool | Description | Use Case |
---|---|---|
TensorFlow | A library for machine learning and deep learning. | Building predictive models that adapt based on learner data. |
Scikit-learn | A machine learning library for data mining and analysis. | Building algorithms to personalize learning content based on learner performance. |
NLTK | A natural language processing library. | Processing and understanding learner's text inputs for adaptive learning interactions. |