R Analysis Course
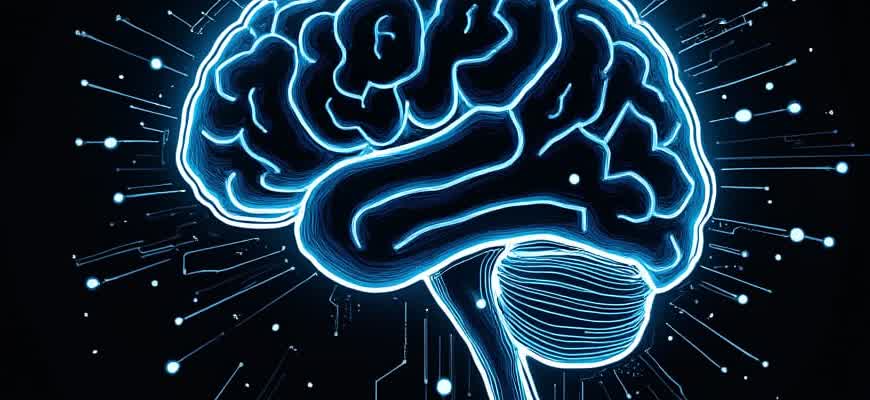
The "R Programming for Data Analysis" course is designed for those who wish to deepen their understanding of statistical computing and data manipulation using R. The course covers a broad range of topics, from basic data structures to advanced data analysis techniques. It is ideal for students, analysts, and researchers who want to enhance their data analysis skills.
Throughout the course, participants will:
- Learn how to use R for data cleaning and transformation.
- Understand the fundamentals of statistical analysis and visualization in R.
- Apply advanced methods like regression, clustering, and machine learning algorithms.
The course structure includes:
- Introduction to R and setting up the environment.
- Data import, export, and management techniques.
- Visualization techniques using ggplot2 and base R.
- Statistical analysis and hypothesis testing.
- Advanced data modeling and machine learning applications.
Important Note: This course assumes no prior programming knowledge, though basic understanding of statistics is recommended. Participants will gain hands-on experience through real-world data analysis projects.
Key topics covered include:
Topic | Description |
---|---|
R Basics | Introduction to syntax, variables, and basic operations in R. |
Data Structures | Vectors, matrices, lists, and data frames in R. |
Data Visualization | Using ggplot2 for effective data visualization. |
Statistical Methods | Exploring hypothesis testing, regression, and ANOVA. |
Getting Started with R for Data Analysis: A Practical Approach
R is a powerful language for data analysis and statistical computing. With its vast collection of packages and libraries, it has become the go-to tool for data scientists, statisticians, and researchers. This guide will help you set up R and start analyzing data efficiently, step by step. Understanding the fundamentals of R will give you a solid foundation to dive deeper into data manipulation, statistical modeling, and visualization.
To begin using R, you first need to install the necessary software and set up your workspace. Afterward, you'll explore basic R syntax, import datasets, and learn how to perform simple data operations. This guide walks you through all essential steps to ensure that you get off to a smooth start.
Step-by-Step Instructions
- Install R and RStudio: Start by downloading and installing the R software from the official R website. Then, install RStudio, a user-friendly interface for R, which provides tools for editing code, managing projects, and visualizing data.
- Set Up Your Working Directory: The working directory is where R looks for files to import and save results. You can set the working directory using the `setwd()` function in R or by using the RStudio interface.
- Install Essential Packages: Use the `install.packages()` function to install packages like dplyr for data manipulation, ggplot2 for visualization, and tidyr for data tidying.
Tip: RStudio has a built-in help system where you can type `?function_name` to get detailed information about any function.
Basic Syntax and Data Operations
Once you've set up R, familiarize yourself with basic syntax for data analysis:
- Variables and Data Types: R uses vectors, matrices, and data frames to store and manipulate data. You can assign values to variables using the `<-` operator, e.g., `x <- 10`.
- Import Data: Use the `read.csv()` function to load datasets in CSV format into R. Once the data is imported, you can begin analyzing it using various functions.
- Data Manipulation: Functions from packages like dplyr allow you to filter, group, and summarize data. For instance, `filter()` helps you select rows based on specific conditions, while `summarize()` calculates summary statistics.
Common Data Analysis Tasks in R
Task | R Function | Description |
---|---|---|
Import Data | read.csv() | Loads CSV files into R as data frames for further analysis. |
Summarize Data | summary() | Generates descriptive statistics (mean, median, etc.) of a dataset. |
Visualize Data | ggplot() | Creates high-quality visualizations like bar charts, scatter plots, etc. |
Note: You can check the documentation of any R function by typing `?function_name` in the console to explore more about how to use it.
Mastering Data Cleaning in R: Key Techniques and Tools
Data cleaning is a critical step in any data analysis process. In R, the rich set of packages and functions offer powerful tools for handling missing values, removing duplicates, and transforming data into the desired structure. Whether you're working with numeric, character, or date-based data, R has a solution to ensure your dataset is ready for analysis.
R provides a wide array of functions and packages like dplyr, tidyr, and data.table to handle various cleaning tasks. Understanding how to use these tools effectively can dramatically improve your workflow and accuracy in any analysis project.
Common Data Cleaning Techniques in R
- Removing Missing Values: Use functions like
na.omit()
ordrop_na()
to remove rows with missing data, or replace missing values with imputation techniques. - Handling Duplicates: Functions like
distinct()
orduplicated()
are essential for identifying and removing duplicate records. - Data Transformation: R provides tools like
mutate()
for creating new variables or modifying existing ones, andseparate()
andunite()
for splitting or combining columns. - Filtering Data: Use
filter()
andselect()
to subset data based on specific conditions or criteria.
Key Packages for Effective Data Cleaning
- dplyr: Offers a set of intuitive functions for data manipulation, such as
filter()
,mutate()
, andarrange()
. - tidyr: Specializes in reshaping and tidying data with functions like
spread()
,gather()
, andseparate()
. - data.table: Provides fast and efficient tools for working with large datasets, especially for subset, join, and aggregation operations.
Important Considerations
Data cleaning is not just about fixing the obvious problems. It requires understanding the context of your dataset and the business problem you're addressing. Each dataset may require a different approach, so it's crucial to adapt your cleaning process to the specific needs of your analysis.
Example: Simple Data Cleaning Workflow
Step | Function | Description |
---|---|---|
1. Remove Missing Values | drop_na() |
Eliminates rows with missing values to prevent errors in analysis. |
2. Remove Duplicates | distinct() |
Removes duplicate rows to ensure unique records. |
3. Transform Data | mutate() |
Creates or modifies columns based on existing data. |
4. Filter Data | filter() |
Subsets data based on specific conditions. |
Building Your First Predictive Model in R: A Step-by-Step Guide
When you're starting out with data analysis in R, building your first predictive model can seem intimidating. However, with the right approach, it becomes a manageable and rewarding experience. A data model in R helps you understand the relationships within your dataset and make predictions based on historical data. This process typically involves data preprocessing, model selection, and evaluation. Below is a practical approach to building your first predictive model using R.
To begin, you need to have a dataset that you can work with. You can either load a sample dataset from R's built-in datasets or use a custom dataset. The process involves several key steps, starting from data inspection and cleaning to model fitting and evaluation. Below is an overview of the essential steps for building a basic predictive model in R.
Steps to Build a Predictive Model in R
- Data Import and Exploration: Load your dataset into R using the read.csv() or read.table() function. Start by exploring the structure of the dataset with functions like str() and summary().
- Data Cleaning: Remove or impute missing values, handle outliers, and ensure data types are consistent.
- Feature Selection: Choose the most relevant features that will contribute to the model's predictive power. This can be done manually or using automated techniques like recursive feature elimination (RFE).
- Model Training: Fit a predictive model, such as a linear regression, decision tree, or random forest, using the lm(), rpart(), or randomForest() functions.
- Model Evaluation: Assess the model's performance by comparing predicted values with actual outcomes. Metrics like RMSE, MAE, or accuracy are commonly used.
Note: Always split your dataset into training and testing sets to avoid overfitting and ensure that your model generalizes well to new data.
Example Code: Building a Linear Regression Model
# Import the dataset data <- read.csv("your_data.csv") # Inspect the dataset str(data) summary(data) # Clean the data (handle missing values, etc.) data_clean <- na.omit(data) # Fit a linear regression model model <- lm(target_variable ~ predictor1 + predictor2, data = data_clean) # Evaluate the model summary(model)
Model Evaluation Metrics
Metric | Description |
---|---|
RMSE (Root Mean Squared Error) | A measure of how well the model's predictions match the actual values. Lower values are better. |
MAE (Mean Absolute Error) | Average of the absolute differences between predicted and actual values. A lower value indicates better model performance. |
Accuracy | For classification models, accuracy measures the percentage of correct predictions. It is not always the best metric for imbalanced datasets. |
By following this process and understanding the key steps, you'll be able to build your first predictive model in R and assess its performance effectively.
Advanced Visualization Techniques in R: Moving Beyond Basic Graphs
Advanced data visualization in R allows for more nuanced and informative graphical representations compared to basic plots. With tools like ggplot2, plotly, and lattice, users can create highly customizable and interactive charts that offer deeper insights. These techniques are especially useful when handling large datasets, complex relationships, or when presenting data to a broader audience who requires clarity and engagement.
Building on the basics, advanced visualizations often involve multi-faceted plots, interactivity, and the ability to represent multidimensional data in an accessible way. Let's explore some of the key techniques and their applications in R for enhanced data presentation.
Advanced Techniques and Their Use Cases
- Heatmaps: Heatmaps are useful for visualizing matrix-like data, where colors represent the magnitude of values. R's pheatmap and ggplot2 can be used to create informative heatmaps that highlight patterns in large datasets.
- Interactive Plots: Using libraries like plotly or shiny, users can create interactive graphs that allow for zooming, hovering, and filtering to explore the data dynamically.
- Faceted Plots: Faceting is an effective technique for visualizing multiple subsets of data on the same plot. ggplot2 makes it easy to create faceted plots, allowing viewers to compare patterns across different categories or time periods.
Key Libraries and Functions
- ggplot2: For highly customizable and aesthetically appealing static plots.
- plotly: For creating interactive plots with hover effects and zoom functionality.
- lattice: A powerful system for creating trellis graphs that are ideal for multivariate data visualizations.
"Advanced visualizations are not just about making pretty pictures; they help reveal hidden patterns and insights that simpler graphs cannot."
Example of a Custom Visualization
Plot Type | Description | R Package |
---|---|---|
Heatmap | Used to visualize the intensity of values across a matrix with color gradients. | ggplot2, pheatmap |
3D Scatter Plot | Displays data in three dimensions, often used for multivariate datasets. | plotly |
Time Series Plot | Used for visualizing trends over time, often with multiple variables. | ggplot2, plotly |
Automating Data Analysis Tasks in R: Streamlining Your Workflow
R provides a powerful environment for data analysis, and automating repetitive tasks is a key strategy to improve efficiency and accuracy in your projects. By leveraging R’s built-in functions and external packages, you can save time and reduce the likelihood of errors. Automation in R allows for the seamless execution of complex tasks, from data cleaning to visualization and statistical modeling.
In this context, automating data analysis tasks involves creating reproducible workflows, which can be executed with minimal manual intervention. By defining reusable functions and employing automation tools like R Markdown or Shiny, you can develop efficient, error-free pipelines that can be used across multiple datasets or projects.
Key Steps to Automate Data Analysis
- Data Preprocessing: Automating the cleaning and transformation of raw data into a usable format.
- Modeling: Using predefined functions to apply statistical models or machine learning algorithms.
- Visualization: Generating charts and graphs automatically based on analysis results.
- Reporting: Creating automated reports to summarize results and insights.
Tools for Streamlining Data Analysis
- R Markdown: Allows for the integration of code, text, and visualizations in one document, making it easy to automate reporting.
- tidyverse: A collection of packages that streamline data manipulation, cleaning, and visualization tasks.
- purrr: A package for applying functions to lists or vectors, making it easy to automate repetitive tasks.
- shiny: A web framework for building interactive applications that automate data processing and visualization.
Example of an Automated Data Analysis Pipeline
Step | Function | Purpose |
---|---|---|
Data Import | read.csv() | Load raw data into R |
Data Cleaning | filter(), mutate() | Remove missing values and transform variables |
Modeling | lm(), glm() | Fit linear or generalized linear models |
Visualization | ggplot2() | Create plots to visualize the results |
Automation is key to maintaining consistent and efficient data analysis processes, saving you time and effort in the long run.
Using R for Predictive Analytics: From Data to Insights
Predictive analytics is an essential aspect of data science that enables businesses and researchers to forecast future outcomes based on historical data. In this process, R plays a crucial role by providing a robust set of tools for data preparation, model development, and evaluation. Leveraging its powerful libraries and flexible syntax, R allows practitioners to transform raw data into actionable insights with ease.
The R programming language offers various statistical techniques for building predictive models. These models can be applied to a wide range of domains, including finance, healthcare, marketing, and more. By implementing the correct algorithms, users can forecast trends, optimize decision-making, and uncover hidden patterns in complex datasets.
Steps for Building Predictive Models in R
- Data Preprocessing: Clean and transform raw data to make it suitable for analysis. This includes handling missing values, scaling variables, and encoding categorical features.
- Model Selection: Choose an appropriate model based on the problem. Common models include linear regression, decision trees, and support vector machines.
- Model Training: Train the model on historical data, adjusting parameters to enhance performance.
- Model Evaluation: Use metrics such as accuracy, precision, and recall to assess the model’s effectiveness.
- Model Deployment: Once the model is fine-tuned, deploy it to make predictions on new, unseen data.
R's versatility and wide range of packages like "caret", "randomForest", and "xgboost" make it an ideal tool for predictive analytics.
Example: Predicting Customer Churn
For example, a company may want to predict which customers are likely to leave. Using R, the analyst could follow these steps:
- Collect and clean customer data (e.g., age, purchase history, customer service interactions).
- Apply a classification model like logistic regression or decision trees to predict churn probability.
- Evaluate model performance with a confusion matrix to ensure accurate predictions.
- Implement the model to proactively target at-risk customers with personalized offers.
Performance Comparison
Model | Accuracy | Precision | Recall |
---|---|---|---|
Logistic Regression | 85% | 80% | 90% |
Random Forest | 88% | 85% | 92% |
Support Vector Machine | 87% | 82% | 89% |
Real-World Applications of R Analysis Across Industries
R is widely recognized for its versatility in data analysis, providing solutions across various sectors. Its powerful statistical capabilities, combined with an extensive set of packages, make it invaluable for professionals in industries ranging from healthcare to finance. Organizations leverage R for data-driven decision-making, predictive modeling, and insights extraction. Here are several key areas where R analysis is applied effectively in real-world scenarios.
In industries such as retail, healthcare, finance, and marketing, R plays a crucial role in optimizing operations, enhancing customer satisfaction, and improving overall efficiency. Below are some of the primary applications in each sector:
Key Applications in Various Sectors
- Healthcare: R is used to analyze clinical trial data, predict patient outcomes, and conduct epidemiological studies.
- Retail: Retailers use R to analyze consumer behavior, track inventory, and develop personalized marketing strategies.
- Finance: In the finance sector, R aids in risk analysis, fraud detection, and portfolio management.
- Marketing: Marketers leverage R to analyze campaign performance, customer segmentation, and social media sentiment analysis.
Detailed Industry-Specific Use Cases
- Healthcare: R’s statistical and machine learning tools allow healthcare professionals to analyze large datasets from patient records and clinical trials. With its ability to process complex datasets, R supports the development of predictive models that help forecast disease outbreaks, patient survival rates, and treatment effectiveness.
- Retail: Retailers use R for predictive analytics to understand consumer purchasing patterns and improve stock management. R’s visualization capabilities also help in tracking sales performance across different store locations and optimizing pricing strategies.
- Finance: R is used extensively in financial modeling, risk management, and fraud detection. For example, R’s time series analysis capabilities allow financial analysts to forecast market trends, while its machine learning tools help identify fraudulent activities in transactions.
- Marketing: R helps marketers analyze large volumes of customer data, including web traffic, social media posts, and purchase history. By using R’s clustering and regression techniques, marketers can identify customer segments, predict future buying behavior, and optimize ad targeting.
Industries and Key Data Analysis Tools in R
Industry | Key R Tools |
---|---|
Healthcare | Survival Analysis, Time Series Forecasting, ggplot2, caret |
Retail | Forecasting, Clustering, dplyr, shiny |
Finance | Quantmod, RiskMetrics, TTR |
Marketing | Sentiment Analysis, Predictive Modeling, text mining, tm |
Important Note: The integration of R into these industries is not just about data analysis; it also enhances the ability to create actionable insights that improve strategic decision-making and drive business growth.