How to Make an Ai Voice Assistant
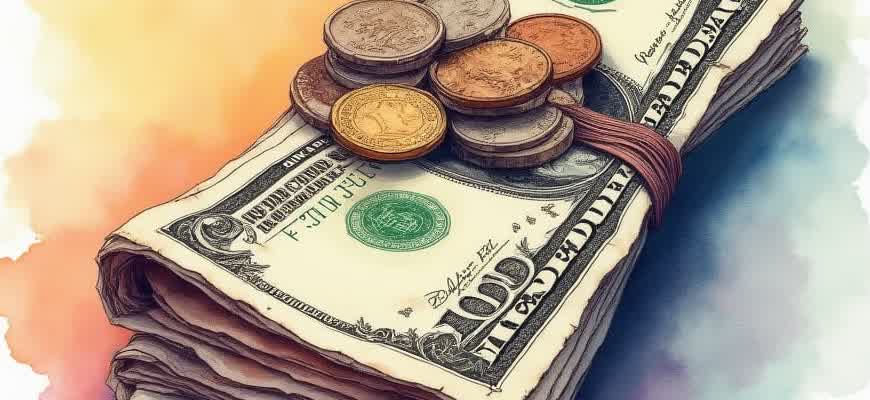
Developing a voice assistant involves several critical steps, including choosing the right tools, programming speech recognition, and integrating AI capabilities. Below is an overview of how to build a functional AI voice assistant from scratch:
- Choosing the Right Platform: Select a programming language and a framework that supports speech processing, like Python with libraries such as SpeechRecognition and PyAudio.
- Speech Recognition: Implement voice input capture and translate audio into text using a speech-to-text engine.
- AI and NLP Integration: Use natural language processing (NLP) libraries, like spaCy or NLTK, to enable the assistant to understand and respond to human queries.
- Voice Output: Integrate text-to-speech (TTS) technology, such as Google Text-to-Speech or pyttsx3, to allow the assistant to speak back to the user.
Once the core components are set, it's essential to integrate various APIs to expand the assistant's functionality. Below is a table outlining some common APIs used in voice assistants:
API | Purpose |
---|---|
Google Cloud Speech API | Speech recognition for converting audio to text. |
Dialogflow | Natural Language Processing (NLP) for understanding user queries. |
Twilio | Voice interaction for making calls and sending messages. |
Note: Testing and optimizing the voice assistant's responses are critical to ensure accuracy and user satisfaction. Regular updates and learning from user interactions help improve the system's performance over time.
How to Build Your Own Voice Assistant
Creating a voice assistant powered by artificial intelligence (AI) requires several components working together: voice recognition, natural language processing (NLP), and speech synthesis. In the process of building your assistant, you will integrate these technologies to allow users to interact with the system using voice commands. The AI will analyze the input, determine the appropriate response, and then speak it back to the user in a natural-sounding voice.
The main tasks involved in the development process are selecting the right frameworks, training the model, and integrating it with speech recognition systems. To make a fully functional voice assistant, it's essential to choose the right tools that align with the goals of your project, whether it's for home automation, customer service, or personal use.
Steps to Create a Voice Assistant
- Choose Speech Recognition Software: Select a platform or library for converting spoken words into text. Popular options include Google's Speech-to-Text API or open-source solutions like CMU Sphinx.
- Natural Language Processing: Use NLP libraries like spaCy or NLTK to analyze and understand the meaning of user queries.
- Text-to-Speech Engine: Integrate a text-to-speech engine, such as Google TTS or Amazon Polly, to convert your assistant’s responses into speech.
- Training Your AI: Train your voice assistant using a set of predefined commands or create custom machine learning models that improve its understanding over time.
- Integration and Deployment: Deploy the voice assistant on your chosen platform, whether it’s a mobile app, smart device, or a web-based interface.
Important Tip: It's crucial to test your assistant under different conditions, including noisy environments, to improve its accuracy and robustness.
Recommended Tools and Frameworks
Tool | Description |
---|---|
Google Cloud Speech API | High-accuracy speech-to-text API that supports various languages. |
spaCy | Advanced NLP library for analyzing text and extracting meaningful data. |
Amazon Polly | Text-to-speech service with lifelike voice synthesis. |
Rasa | Open-source conversational AI framework for creating voice assistants. |
Choosing the Right Technology Stack for Your Voice AI Assistant
When building a voice assistant, selecting the appropriate technology stack is critical for ensuring the efficiency, scalability, and flexibility of the system. Your choice of technologies will define how well your assistant can handle tasks like natural language processing (NLP), speech recognition, and real-time responses. In this process, it is important to focus on specific components such as voice recognition, cloud infrastructure, and APIs that offer seamless integration with the desired platforms.
There are several key considerations when selecting technologies for a voice assistant. The stack should not only meet current requirements but also allow for future growth, with the ability to incorporate new features and improve performance over time. Below are essential components and tools to evaluate when choosing the right stack for your project.
Key Components of a Voice Assistant Technology Stack
- Speech Recognition: This technology converts spoken language into text. Common libraries include Google's Speech-to-Text, IBM Watson, and Microsoft Azure's Speech API.
- Natural Language Processing (NLP): NLP helps in understanding and processing the meaning behind user inputs. Popular frameworks include spaCy, NLTK, and Hugging Face's Transformers.
- Text-to-Speech (TTS): A TTS engine converts text responses into human-like speech. Options include Google Cloud Text-to-Speech, Amazon Polly, and Microsoft Azure's TTS.
- Dialog Management: This is the core part of voice assistant architecture, responsible for managing multi-turn conversations. Frameworks like Rasa and Botpress are widely used for this purpose.
Considerations for Cloud and Infrastructure
Cloud services are often chosen to handle the computational load and storage needs of voice assistants. Here are some considerations:
- Scalability: Choose cloud platforms that can easily scale with increasing numbers of users and data, such as AWS, Google Cloud, or Azure.
- Latency: A low-latency system is crucial for providing real-time responses. Edge computing or local servers might be necessary for sensitive applications.
- Security: Ensure that data is encrypted and that the system complies with privacy regulations like GDPR.
Evaluation Table: Popular Tech Choices
Technology | Use Case | Best For |
---|---|---|
Google Cloud Speech-to-Text | Speech Recognition | Fast, high-quality transcription |
AWS Polly | Text-to-Speech | Natural-sounding voices |
Rasa | Dialog Management | Open-source, customizable bots |
Tip: When selecting a technology stack, always consider the specific needs of your application. The stack should align with the target platforms and user expectations for performance and quality.
Integrating Speech Recognition: Best Tools and Libraries
Speech recognition is a crucial component in the development of AI voice assistants. It allows the system to convert spoken language into text, enabling interaction with users through voice commands. In this section, we explore some of the best tools and libraries that can facilitate the integration of speech recognition in voice assistants.
When selecting a speech recognition tool or library, it is important to consider factors such as accuracy, language support, ease of integration, and resource consumption. Below are several top options that are widely used in the industry for building robust speech recognition systems.
Top Speech Recognition Tools and Libraries
- Google Cloud Speech-to-Text: A cloud-based API offering high accuracy, support for multiple languages, and real-time transcription capabilities.
- Microsoft Azure Speech Service: Provides both speech recognition and natural language processing. It supports custom models and integrates easily with other Azure services.
- CMU Sphinx (PocketSphinx): An open-source toolkit that is particularly useful for offline voice recognition applications, though it may have slightly lower accuracy compared to cloud-based solutions.
- SpeechRecognition Library (Python): A Python library that wraps several speech recognition APIs, including Google Web Speech API, Microsoft Bing Voice Recognition, and more. It's suitable for quick implementation and testing.
Choosing the Right Tool: Key Considerations
- Accuracy: Cloud-based services like Google and Microsoft tend to have the highest accuracy, but open-source tools can still be suitable for certain applications.
- Customization: If you need specific features or domain-specific models, some libraries like Microsoft Azure provide customization options.
- Latency and Resource Usage: Real-time applications may require low-latency processing. Local libraries like CMU Sphinx are better suited for resource-constrained environments.
- Language Support: Ensure the tool you choose supports the languages you need. Google Cloud and Microsoft Azure support a wide variety of languages and accents.
Comparison of Popular Speech Recognition Libraries
Library | Type | Supported Languages | Offline Support | Customization |
---|---|---|---|---|
Google Cloud Speech-to-Text | Cloud API | Multiple | No | Limited |
Microsoft Azure Speech | Cloud API | Multiple | No | High |
CMU Sphinx | Offline | Limited | Yes | Moderate |
SpeechRecognition Library (Python) | Wrapper (Multiple) | Multiple | Depends on API | Low |
When integrating speech recognition, consider the specific needs of your voice assistant, such as language support and whether offline capabilities are necessary.
Designing Natural Language Understanding (NLU) for Voice Commands
When developing an AI voice assistant, creating a robust Natural Language Understanding (NLU) system is crucial for interpreting and processing voice commands accurately. NLU is responsible for transforming spoken language into a structured format that the system can act upon. It involves multiple steps, including speech recognition, intent classification, and entity extraction. Each of these processes plays a key role in understanding the user's request and providing an appropriate response.
The core challenge in NLU design is ensuring that the system can handle a wide range of phrases and words with varying syntax. This is essential because voice commands are often informal, with users expressing themselves in different ways. Therefore, the assistant must recognize synonyms, paraphrases, and complex sentence structures to offer meaningful responses.
Steps for Building an Effective NLU System
- Speech Recognition: Convert audio input into text using speech-to-text models.
- Intent Detection: Identify the user's intention behind the command, such as setting a reminder or checking the weather.
- Entity Recognition: Extract specific details (e.g., date, time, location) from the command.
- Context Understanding: Retain context across multiple interactions to handle follow-up commands effectively.
"Effective NLU systems must adapt to diverse user speech patterns and ensure high accuracy in interpreting varying commands."
Challenges in NLU Implementation
- Ambiguity Handling: Users may provide unclear or ambiguous commands. For example, "Set an alarm" can be interpreted in many ways based on context.
- Multilingual Support: Handling different languages or dialects can complicate the extraction of intent and entities.
- Naturalness vs Precision: Striking a balance between understanding natural language input and ensuring precision in command interpretation is difficult.
NLU System Performance Metrics
Metric | Description | Goal |
---|---|---|
Accuracy | The percentage of correctly interpreted voice commands. | Minimize errors in intent detection and entity extraction. |
Latency | The time taken to process and respond to a voice command. | Ensure quick responses, typically under a few seconds. |
Coverage | The range of possible user intents and entities the system can handle. | Expand to cover as many scenarios and commands as possible. |
How to Train Your AI Assistant with Custom Data
Training an AI assistant with tailored data allows you to create a more responsive and personalized system. By leveraging domain-specific datasets, you can refine the assistant's ability to handle unique tasks, understand specialized terminology, and interact with users more effectively. Whether you're focusing on customer support, a niche industry, or a particular language, using custom data is essential for improving the accuracy of your AI assistant.
To train an AI assistant, you'll need to prepare datasets that reflect the real-world scenarios your assistant will face. These datasets often include text-based conversations, commands, and questions that the assistant must be able to understand and respond to. The quality and relevance of the data are crucial for achieving effective performance.
Steps to Train Your AI Assistant with Custom Data
- Data Collection: Gather conversations, questions, and tasks relevant to your assistant's domain. These can be sourced from existing customer interactions, industry-specific documents, or manually created datasets.
- Data Preprocessing: Clean and format the data to remove irrelevant information, fix typos, and structure it for optimal training. This includes tokenizing sentences, removing stop words, and standardizing formats.
- Labeling and Annotation: Annotate the data with labels such as intents (e.g., "order status", "weather forecast") and entities (e.g., "product name", "location") to help the assistant identify key information in user inputs.
- Model Training: Use machine learning algorithms, such as Natural Language Processing (NLP), to train the assistant using the annotated data. Iteratively refine the model by testing its performance and adjusting parameters.
- Continuous Improvement: Continuously feed new data into the system and retrain the model to ensure it adapts to changes in user behavior and domain-specific requirements.
Best Practices for Data Preparation
- Data Diversity: Ensure your dataset includes a wide variety of user inputs, covering different phrasing and scenarios.
- Quality Over Quantity: Prioritize the quality of the data over the sheer volume. Clean, accurate data will yield better results than large, noisy datasets.
- Realistic Context: Use real-world interactions as much as possible to help the assistant understand natural conversation patterns.
Sample Dataset Structure
Intent | Example Phrase | Entities |
---|---|---|
Order Status | What is the status of my order? | Order Number |
Weather Forecast | What's the weather like tomorrow? | Location |
Product Inquiry | Tell me more about the iPhone 13 | Product Name |
Tip: Always validate the assistant's responses by testing with real users and collecting feedback to improve the model's performance.
Implementing Text-to-Speech (TTS) for Clear Communication
Text-to-speech (TTS) technology is an essential component of AI voice assistants, allowing them to communicate with users through natural-sounding speech. Proper integration of TTS ensures that the voice assistant's responses are both intelligible and pleasant to hear, improving the user experience. The clarity and fluidity of the synthesized voice are crucial for effective interaction, especially in environments where users may rely heavily on auditory information.
To achieve this, TTS systems utilize advanced algorithms that convert written text into spoken words. These systems typically support various languages, accents, and voices, making them adaptable to different user preferences. By selecting the appropriate TTS engine and fine-tuning its parameters, developers can create a voice assistant that sounds natural and delivers clear, context-aware responses.
Key Steps in Implementing TTS for Optimal Communication
- Choose an appropriate TTS engine that supports multiple languages and customization options.
- Optimize speech clarity by adjusting speech speed, pitch, and volume levels.
- Ensure that the TTS engine can handle diverse content, such as technical terms or varied sentence structures.
- Test the system with real users to identify areas for improvement in pronunciation and intonation.
Tip: Choose a TTS engine with natural-sounding voices to avoid robotic or artificial tones that could detract from the user experience.
Factors Affecting TTS Output Quality
Factor | Impact on TTS |
---|---|
Voice Selection | Different voices can significantly affect user perception, with some sounding more natural than others. |
Speech Rate | Faster speech may sound rushed, while slower speech can seem unnatural. The rate should be balanced based on user preferences. |
Pronunciation | Correct pronunciation of words, especially those with non-standard spellings, is critical for user comprehension. |
Important: Fine-tuning the TTS system to match the target audience’s language proficiency and preferences will enhance overall clarity and understanding.
Ensuring Privacy and Security in AI Voice Assistants
When developing AI-powered voice assistants, maintaining user privacy and data security is critical. As these assistants process sensitive information, it is essential to implement measures that protect users' personal data and ensure secure interactions. One of the primary concerns is the continuous listening feature of voice assistants, which may inadvertently collect private conversations or personal information without consent. Developers must integrate robust mechanisms to address these issues, ensuring transparency and control for the users.
Security protocols must cover both data storage and transmission to safeguard against unauthorized access. This involves employing end-to-end encryption and anonymizing user data wherever possible. Additionally, voice assistants should include clear options for users to manage their data, including viewing, modifying, and deleting information stored by the assistant.
Key Privacy Measures for Voice Assistants
- Data Encryption: All communication between the user and the voice assistant should be encrypted, ensuring that unauthorized parties cannot access sensitive information.
- User Consent: Voice assistants should always ask for explicit permission before collecting or storing personal data.
- Local Processing: Whenever possible, voice assistants should process data locally on the user's device, reducing the amount of data transmitted to external servers.
Security Best Practices for Voice Assistant Development
- Authentication Mechanisms: Incorporate biometric or multi-factor authentication to ensure that only authorized users can interact with the assistant.
- Regular Security Audits: Conduct frequent security assessments to identify and address potential vulnerabilities in the voice assistant's system.
- Data Minimization: Limit the collection and storage of personal data to only what is necessary for the voice assistant's functionality.
"A voice assistant should act as a trusted partner, respecting privacy and ensuring security while providing value through personalized interactions."
Security Data Handling
Data Type | Security Measure |
---|---|
Voice Recordings | Encrypted storage and anonymization |
Personal Data | Explicit user consent and minimal retention |
Communication Channels | End-to-end encryption |
Optimizing Performance for Real-Time Voice Interaction
When building an AI voice assistant, ensuring smooth and fast response times during real-time interaction is crucial for user satisfaction. Achieving this requires optimizing various components of the system, from speech recognition to response generation. Latency reduction is one of the most significant factors to consider when designing a system that can interact effectively in real-time.
There are multiple techniques to optimize performance in real-time voice assistants, from leveraging efficient algorithms to choosing the right hardware. This process involves reducing computational complexity, optimizing speech-to-text and text-to-speech models, and fine-tuning the neural network for faster responses.
Key Areas for Optimization
- Efficient Speech Recognition – Streamlining the process by using optimized models that can quickly process incoming audio signals and convert them into text.
- Optimized Response Generation – Ensuring that the natural language processing (NLP) models are light enough to generate responses in real-time without significant delay.
- Hardware Considerations – Utilizing edge devices or cloud computing resources that offer low latency for processing voice data quickly.
Reducing latency and computational load during the interaction is essential for a seamless experience in real-time voice assistants.
Optimization Techniques
- Model Pruning – This technique involves removing unnecessary parts of the model to reduce the size and computational requirements while maintaining the quality of performance.
- Edge Computing – Processing data locally on the device, such as smartphones or smart speakers, instead of relying solely on cloud-based services.
- Optimizing Audio Preprocessing – Enhancing audio quality before it reaches the recognition system to reduce noise and improve the accuracy of speech recognition.
Performance Metrics
Metric | Goal |
---|---|
Latency | Under 100 milliseconds for real-time interaction |
Speech Recognition Accuracy | Above 95% accuracy in diverse environments |
Response Time | Less than 500 milliseconds for generating a response |