Program Ai Tutorial
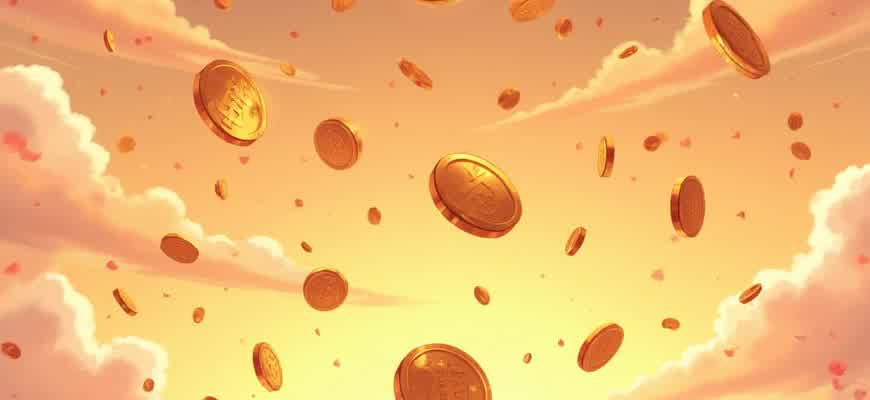
Artificial Intelligence (AI) development requires a solid understanding of both algorithms and the tools used to implement them. In this tutorial, we will explore the essential steps and concepts involved in creating AI applications, from initial setup to advanced techniques.
Key Steps to Begin
- Understanding the basic AI concepts
- Choosing the right programming language and tools
- Familiarizing with machine learning algorithms
- Implementing simple AI models
Core AI Concepts
Concept | Description |
---|---|
Machine Learning | A method of data analysis that automates analytical model building. |
Neural Networks | Algorithms inspired by the structure of the human brain, used for pattern recognition. |
Natural Language Processing | Techniques used to process and analyze human language data. |
"AI development requires not only theoretical knowledge but also hands-on experience with tools and libraries like TensorFlow, PyTorch, and Scikit-Learn."
How to Begin with AI Development: A Step-by-Step Approach
Entering the world of artificial intelligence (AI) programming can be overwhelming, but breaking it down into clear steps can make the process much more manageable. First, it’s essential to understand the fundamentals of AI, machine learning, and deep learning before diving into coding. Once you have a solid theoretical foundation, you can move on to practical implementation using various programming languages and tools. This guide will help you get started in the most efficient way possible.
The key to success in AI programming is persistence and continuous learning. It’s important to start small and gradually increase the complexity of the projects you work on. Over time, as you become more comfortable with the concepts, you can experiment with more advanced AI models and techniques. Here’s a step-by-step approach to help you launch your journey in AI programming.
Steps to Begin AI Programming
- Learn the Basics of Programming: Before tackling AI, make sure you have a strong grasp of programming concepts. Python is the most commonly used language in AI development, but others like R, Java, and C++ are also important.
- Familiarize yourself with syntax and data structures in Python.
- Understand how functions, loops, and conditional statements work.
- Understand the Core Concepts of AI: Learn about machine learning, neural networks, and algorithms that power AI systems.
- Study linear algebra, probability theory, and statistics.
- Get familiar with supervised and unsupervised learning techniques.
- Set Up Your Development Environment: Install necessary tools and libraries like TensorFlow, PyTorch, NumPy, and SciPy.
- Install Anaconda for easy package management.
- Set up Jupyter Notebook or any IDE for coding.
- Start with Simple Projects: Begin with small, manageable projects like image classification, chatbots, or basic recommendation systems to build practical experience.
- Use datasets from Kaggle to train and test your models.
- Experiment with existing algorithms and tweak them for your needs.
"The key to mastering AI is to start small, learn continuously, and apply your knowledge through real-world projects." - AI Expert
Key Tools and Libraries to Get Started
Library | Description |
---|---|
TensorFlow | A popular library for creating and training deep learning models. |
PyTorch | Another widely-used deep learning framework with a focus on flexibility. |
Scikit-learn | Provides simple tools for data mining and machine learning algorithms. |
Keras | High-level neural networks API, running on top of TensorFlow. |
Choosing the Right Tools for AI Development: What You Need to Know
When it comes to building AI applications, selecting the right tools can be a make-or-break decision. The choices are vast, ranging from programming languages to frameworks and libraries. Understanding the strengths and weaknesses of these tools will help you streamline your development process and create more efficient solutions. In this guide, we will explore essential aspects to consider when choosing AI development tools, such as language selection, libraries, and frameworks.
Several factors contribute to the success of an AI project. These include scalability, compatibility with your project’s goals, community support, and ease of use. By evaluating these factors, you can decide which combination of tools will best suit your needs. Below, we discuss key tools and technologies that every AI developer should be familiar with.
Key Factors to Consider
- Programming Language: Python is the most widely used language for AI due to its simplicity and vast number of libraries. However, R and Java also offer unique advantages, especially for statistical analysis and large-scale enterprise solutions.
- Frameworks and Libraries: Frameworks like TensorFlow and PyTorch dominate the deep learning landscape, while libraries such as scikit-learn are excellent for machine learning tasks.
- Hardware Compatibility: High-performance computing often requires specialized hardware, such as GPUs. Ensure that the tools you choose can fully leverage hardware acceleration.
Popular AI Tools at a Glance
Tool | Use Case | Strength |
---|---|---|
TensorFlow | Deep Learning | Scalability and production readiness |
PyTorch | Deep Learning | Flexibility and ease of use for research |
scikit-learn | Machine Learning | Comprehensive algorithms and simple API |
OpenCV | Computer Vision | Real-time image processing |
Important: Always assess the community support for a given tool. A large, active community ensures quicker problem-solving and access to more learning resources.
Choosing the Right Combination
- Project Type: Determine whether your project is focused on computer vision, natural language processing, or predictive analytics. This will influence your framework choice.
- Team Expertise: Choose tools that align with the skills of your team. Using tools that your team is already familiar with will reduce development time and increase efficiency.
- Scalability Requirements: Ensure the tools you select can scale with your project as it grows. Some libraries are more suited for small-scale applications, while others handle large datasets better.
Building Your First AI Model: Practical Examples and Tips
Creating an AI model can seem daunting at first, but breaking down the process into manageable steps makes it much easier. Whether you're developing a model for classification, regression, or any other task, understanding the core workflow is crucial. This guide will walk you through practical examples of how to build your first AI model, along with useful tips to help you get started.
Before diving into coding, it's important to grasp the fundamentals. The most basic steps in building an AI model typically involve data collection, preprocessing, model selection, training, and evaluation. Let’s explore each stage with examples and actionable advice to help you successfully build your model from scratch.
Steps to Build Your First Model
- Step 1: Gather and Prepare Data
- Step 2: Select the Right Algorithm
- Step 3: Train Your Model
- Step 4: Evaluate and Improve
Start by collecting data that is relevant to the problem you're solving. This data will need to be preprocessed to ensure it's clean and suitable for model training. Techniques like normalization, missing value imputation, and feature encoding might be necessary.
Choosing the appropriate machine learning algorithm depends on the problem you're trying to solve. For classification tasks, algorithms like Logistic Regression, Decision Trees, or Neural Networks may be appropriate. For regression, Linear Regression or Random Forest are common choices.
Split your data into training and testing sets. Use the training set to train your model and the test set to evaluate its performance. Monitor performance metrics like accuracy, precision, recall, and F1 score to assess how well your model is performing.
Once your model has been trained, it's essential to evaluate its performance. If it doesn't perform well, try adjusting hyperparameters, using different features, or choosing another algorithm. Model tuning is an iterative process.
Practical Example: Classifying Images with a Neural Network
Let's say you want to build an AI model to classify images of animals. Here's an outline of how to do it:
- Gather a dataset of labeled animal images.
- Preprocess the images (resize, normalize pixel values).
- Split the dataset into training and testing sets.
- Build a Convolutional Neural Network (CNN) model using a framework like TensorFlow or PyTorch.
- Train the model on the training set and evaluate using the test set.
- Tune the model by adjusting the number of layers or neurons in the CNN.
Key Tips for Building an AI Model
Tip | Description |
---|---|
Data Quality is Crucial | Make sure your data is clean, labeled accurately, and representative of real-world scenarios. |
Start Simple | Begin with a basic model and gradually build complexity. This will help you understand the fundamentals before tackling advanced techniques. |
Monitor Overfitting | Regularly check for overfitting by testing your model on unseen data. Use techniques like cross-validation and early stopping to prevent it. |
Important: Always ensure that your model's performance is evaluated on data it hasn't seen during training. This helps to check its generalization capability.
Data Preparation for Artificial Intelligence: Essential Techniques to Master
Data preprocessing is a crucial step in the AI model development pipeline. It involves transforming raw data into a format that is more suitable for analysis and model training. Without proper data preparation, even the most sophisticated machine learning algorithms can struggle to produce accurate results. This step is where you define how your data should be cleaned, structured, and transformed to maximize the effectiveness of your AI systems.
Mastering the techniques of data preprocessing ensures that you are optimizing the input for your models. While there are numerous approaches, certain methods are universally essential across different types of AI projects. Understanding how and when to use these techniques can dramatically improve model performance and reliability.
Key Data Preprocessing Techniques
- Handling Missing Data: Many datasets contain missing values, which can distort your analysis. Approaches include:
- Imputation (replacing missing values with the mean, median, or mode)
- Deletion (removing rows or columns with missing values)
- Using algorithms that can handle missing data directly
- Normalization and Standardization: Scaling the data helps ensure that features contribute equally to the model. Techniques include:
- Min-Max Scaling (rescaling features to a [0,1] range)
- Z-score Standardization (transforming features to have a mean of 0 and a standard deviation of 1)
- Data Encoding: Categorical variables need to be converted into numerical values. Common methods are:
- One-Hot Encoding (creating binary columns for each category)
- Label Encoding (assigning a unique integer to each category)
- Outlier Detection: Identifying and removing outliers ensures that extreme values do not disproportionately influence your model. Techniques include:
- IQR (Interquartile Range) method
- Z-score method
Effective preprocessing is not just about cleaning the data; it’s about optimizing it for the model you plan to use. Choose your techniques based on the data characteristics and the machine learning algorithm's requirements.
Practical Example: A Simple Data Cleaning Table
Feature | Raw Data | Preprocessed Data |
---|---|---|
Age | 25, 30, NULL, 35, 45 | 25, 30, 35, 35, 45 (Imputed) |
Income | 3000, 4000, 5000, NULL, 7000 | 3000, 4000, 5000, 5000 (Imputed) |
Gender | Male, Female, Female, Male, Male | 0, 1, 1, 0, 0 (Label Encoded) |
How to Train Your AI Model with Real-World Data
Training an AI model using real-world data is crucial to ensure that the model performs effectively in real-life scenarios. Unlike synthetic datasets, real-world data can be messy, incomplete, or biased, making it essential to follow a structured approach. Below are the key steps to train your AI model using actual data.
The process starts with data collection, followed by preprocessing, model selection, training, and fine-tuning. By leveraging real-world data, your model can better generalize and make accurate predictions. The following sections will guide you through each step in detail.
Key Steps to Train Your AI Model
- Data Collection: Gather data that reflects the problem you are solving. This can include structured data (e.g., databases) or unstructured data (e.g., images, text).
- Data Cleaning: Remove any irrelevant, noisy, or incomplete data that could negatively impact the model’s performance.
- Feature Engineering: Extract meaningful features from raw data to make it easier for the AI model to understand patterns.
- Model Selection: Choose an appropriate model based on the type of data and problem you are addressing (e.g., regression, classification, or deep learning).
- Training: Use the prepared data to train the model, adjusting parameters to minimize errors.
- Evaluation: Assess the model's performance on a separate validation set to ensure it generalizes well to new data.
- Fine-tuning: After training, tweak the model’s hyperparameters and retrain it if necessary for better accuracy.
Real-World Data Challenges
"Real-world data is often messy and unpredictable, but with the right approach, it can be harnessed to create highly accurate models."
One major challenge when working with real-world data is handling missing values, which can occur due to errors during data collection. It’s essential to fill or remove these values without introducing bias into the model. Additionally, the data might be unbalanced, where some classes are underrepresented. Addressing this imbalance through techniques like oversampling or using specialized loss functions can improve model accuracy.
Example Table: Data Preprocessing Overview
Step | Task | Tools/Methods |
---|---|---|
Data Collection | Gather relevant real-world data | Web scraping, APIs, public datasets |
Data Cleaning | Remove duplicates, handle missing values | Python (Pandas, NumPy), data imputation |
Feature Engineering | Transform raw data into useful features | Data normalization, one-hot encoding |
Assessing the Performance of AI Models: Key Metrics and Methods You Can Use Now
When evaluating the performance of artificial intelligence models, it's essential to apply a range of metrics to understand how well they meet specific requirements. These metrics help in making decisions about which models are most effective for a given task, ensuring they deliver expected results. Commonly used measures include accuracy, precision, recall, and F1-score, but the choice depends on the task and data available.
Model evaluation also requires a systematic approach to testing and comparison. This involves applying different evaluation methods that can help highlight areas of improvement. Key techniques include cross-validation, confusion matrices, and learning curves. By using these tools, developers can better understand their model's strengths and weaknesses, making it easier to iterate and improve its performance.
Metrics to Track
- Accuracy: Measures the overall correctness of the model, calculated as the ratio of correct predictions to total predictions.
- Precision: Indicates the proportion of true positive predictions among all positive predictions.
- Recall: Reflects the proportion of true positives identified out of all actual positives in the dataset.
- F1-score: The harmonic mean of precision and recall, giving a balance between the two metrics.
- AUC-ROC: Evaluates the model’s ability to distinguish between classes at different thresholds, plotted on a Receiver Operating Characteristic curve.
Methods for Evaluation
- Cross-validation: Involves splitting the dataset into multiple subsets to train and test the model repeatedly. This helps mitigate overfitting and provides a more reliable estimate of the model's performance.
- Confusion Matrix: A table that displays the number of correct and incorrect predictions broken down by class. It helps identify false positives and false negatives.
- Learning Curves: Graphs that show how the model's performance improves over time as it is trained with more data or iterations.
Key Takeaways
It’s important to choose the right combination of metrics and methods for evaluating your AI model based on the task at hand. For example, if the dataset is highly imbalanced, metrics like precision, recall, and F1-score can be more informative than accuracy.
Example of Confusion Matrix
Actual \ Predicted | Positive | Negative |
---|---|---|
Positive | True Positive (TP) | False Negative (FN) |
Negative | False Positive (FP) | True Negative (TN) |
Deploying AI Models: A Practical Approach to Making Your Model Accessible
When you have developed an AI model, the next crucial step is making it available to users or integrating it into existing systems. This phase can be challenging, as it involves ensuring that your model is both reliable and scalable, while also offering easy access for your target audience. The deployment process involves several stages, from selecting a hosting environment to managing the model's performance post-deployment.
Effective deployment is about more than just hosting a model; it includes optimizing performance, ensuring security, and providing a user-friendly interface. In this guide, we’ll cover the essential steps to deploy AI solutions, from choosing infrastructure to setting up APIs for interaction.
Key Steps to Deploying AI Models
- Choosing the Right Hosting Environment:
Before deploying, you need to choose a suitable environment based on your model's needs, such as cloud services, on-premise servers, or edge devices. Popular cloud platforms include AWS, Google Cloud, and Microsoft Azure, which provide scalable solutions for AI models.
- Model Optimization:
Optimizing your model involves reducing latency and improving the response time. Techniques like model compression, quantization, and pruning can significantly improve performance without sacrificing accuracy.
- Creating APIs for Access:
APIs allow users to interact with the model. RESTful APIs are common for deploying machine learning models, enabling easy communication between the client and the server.
Best Practices for AI Model Deployment
- Monitoring Performance: Continuously monitor the model's performance after deployment to ensure it meets expected outcomes. Tools like Prometheus and Grafana can be used for real-time monitoring.
- Ensuring Security: Implement strong authentication and encryption protocols to protect sensitive data and prevent unauthorized access.
- Scalability: Plan for scaling your model to handle increased demand, whether through auto-scaling in the cloud or distributing workloads across multiple servers.
Deployment Platforms Comparison
Platform | Key Features | Best For |
---|---|---|
AWS SageMaker | Fully managed service for building, training, and deploying models | Large-scale models requiring powerful infrastructure |
Google AI Platform | Supports TensorFlow and other frameworks, seamless integration with Google Cloud | Deep learning models and integration with Google services |
Microsoft Azure ML | Comprehensive suite for model training and deployment, integration with Azure | Enterprises using other Microsoft services |
“Deployment is not the end of the journey; it's where continuous improvement begins.”